Lightning web components (LWC) interview questions
Can you explain the difference between @track and @api decorators?
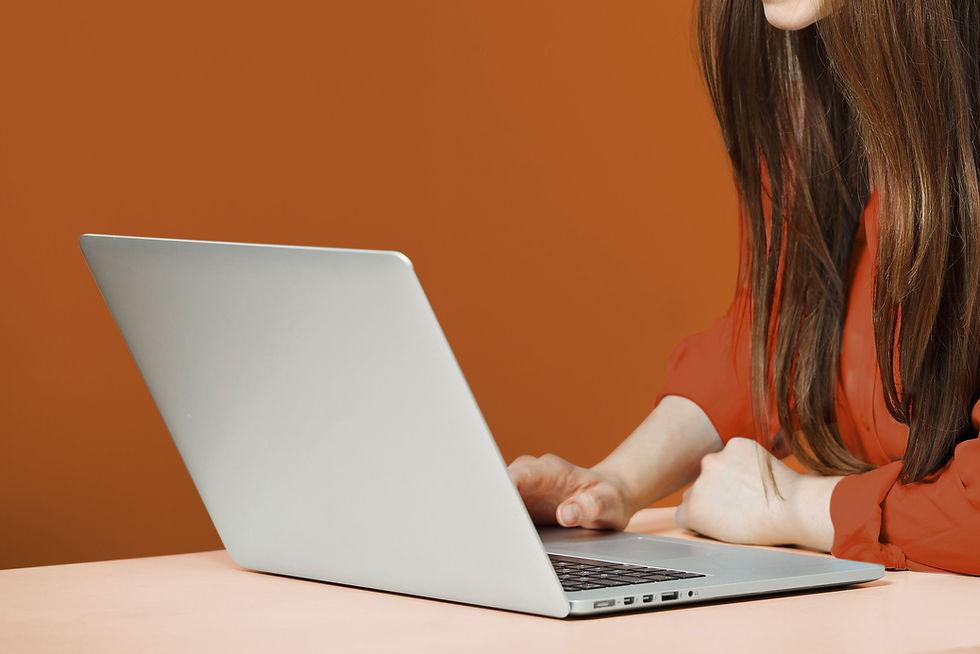
Salesforce Lightning Web Components (LWC) is a modern programming model for building enterprise-grade web applications. It provides developers with a set of reusable components and tools for building responsive user interfaces.
One of the key features of LWC is its use of decorators, which are special keywords decorators in LWC: @api, @wire, and @track.
@api
The @api decorator is used to expose a public property or method on a LWC component. This allows other components to access and interact with the decorated property or method.
For example, consider the following HelloWorld component that uses the @api decorator to expose a message property:
import { LightningElement, api } from 'lwc';
export default class HelloWorld extends LightningElement {
@api
message = 'Hello World!';
}
This message property can now be accessed and updated by other components that use the HelloWorld component. For example, the following component could be used to update the message property of the HelloWorld component:
import { LightningElement } from 'lwc';
export default class UpdateMessage extends LightningElement {
handleClick() {
// Get a reference to the HelloWorld component
const helloWorld = this.template.querySelector('c-hello-world');
// Update the message property of the HelloWorld component
helloWorld.message = 'Hello Salesforce!';
}
}
@wire
The @wire decorator is used to wire a property or method in a LWC component to a data source. This allows the component to automatically receive data from the specified data source, without having to manually fetch or subscribe to the data.
For example, consider the following AccountList component that uses the @wire decorator to wire its accounts property to the @salesforce/apex/AccountController.getAccounts Apex method:
import { LightningElement, wire } from 'lwc';
import getAccounts from '@salesforce/apex/AccountController.getAccounts';
export default class AccountList extends LightningElement {
@wire(getAccounts)
accounts;
// Other component logic...
}
This accounts property will now automatically receive a list of accounts from the getAccounts Apex method whenever the component is rendered or the data changes. The component does not need to manually call the Apex method or subscribe to any data change events.
@track
The @track decorator is used to track changes to a property in a LWC component. This allows the component to automatically rerender itself whenever the value of the decorated property changes.
For example, consider the following Counter component that uses the @track decorator to track changes to its count property:
import { LightningElement, track } from 'lwc';
export default class Counter extends LightningElement {
@track
count = 0;
increment() {
this.count++;
}
decrement() {
this.count--;
}
}
Whenever the increment or decrement method is called, the count property will be updated, and the component will automatically rerender itself to reflect the new value. This allows the component to always display the current value of the count property, without having to manually update the UI. https://blog.christianpelayo.com/leveraging-the-power-of-decorators-in-your-lwc-components
Comments