ParentComponent and ChildComponent. The parent component needs to pass data to the child, and the child component should notify the parent when an action is performed.
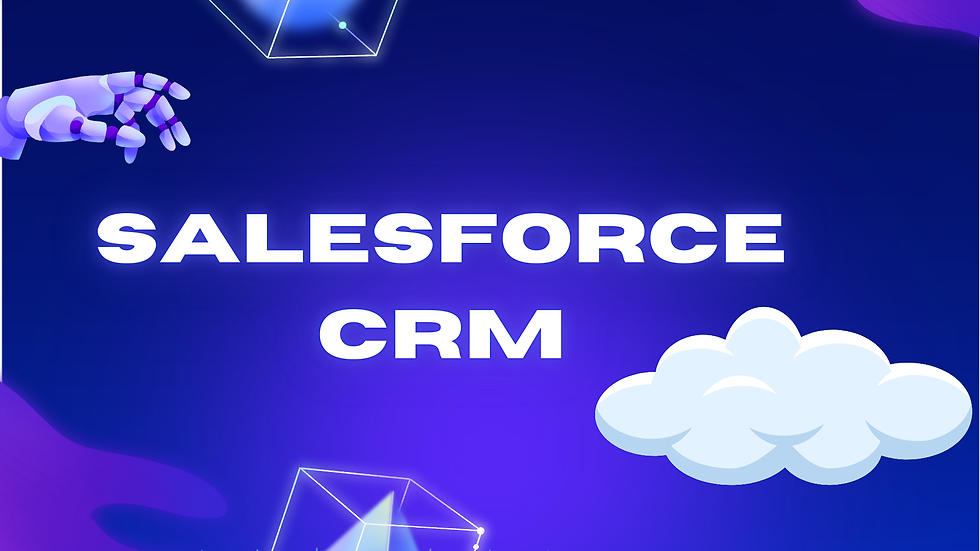
Question: How would you pass data from the parent to the child component? How can the child notify the parent component when an event occurs?
To pass fields like `Name` and `Id` from the Account component (parent) to the Contact component (child), and allow the child to notify the parent when an event occurs, follow these steps:
Parent Component (Account)
The parent component will:
1. Pass the `Name` and `Id` fields as props to the child component.
2. Handle an event dispatched from the child.
AccountComponent.html:
```html
<template>
<lightning-card title="Account Component">
<p class="slds-p-horizontal_small">
Account Name: {accountName}, Account Id: {accountId}
</p>
<c-contact-component
account-name={accountName}
account-id={accountId}
oncontactevent={handleContactEvent}>
</c-contact-component>
</lightning-card>
</template>
```
AccountComponent.js:
```javascript
import { LightningElement } from 'lwc';
export default class AccountComponent extends LightningElement {
accountName = 'Acme Corporation';
accountId = '001XXXXXXXXXXXXXXX';
handleContactEvent(event) {
const { contactName, contactId } = event.detail;
console.log(`Received from Contact: ${contactName}, ${contactId}`);
alert(`Contact updated: Name - ${contactName}, Id - ${contactId}`);
}
}
```
---
Child Component (Contact)
The child component will:
1. Receive `accountName` and `accountId` as props.
2. Dispatch an event to the parent with data when a button is clicked.
ContactComponent.html:
```html
<template>
<lightning-card title="Contact Component">
<p class="slds-p-horizontal_small">
Received Account Name: {accountName}, Account Id: {accountId}
</p>
<lightning-input type="text" label="Contact Name" value={contactName} onchange={handleNameChange}></lightning-input>
<lightning-input type="text" label="Contact Id" value={contactId} onchange={handleIdChange}></lightning-input>
<lightning-button label="Notify Account" onclick={notifyParent}></lightning-button>
</lightning-card>
</template>
ContactComponent.js:
```javascript
import { LightningElement, api } from 'lwc';
export default class ContactComponent extends LightningElement {
@api accountName; // Received from parent
@api accountId; // Received from parent
contactName = '';
contactId = '';
handleNameChange(event) {
this.contactName = event.target.value;
}
handleIdChange(event) {
this.contactId = event.target.value;
}
notifyParent() {
const event = new CustomEvent('contactevent', {
detail: {
contactName: this.contactName,
contactId: this.contactId,
}
});
this.dispatchEvent(event);
}
}
How It Works
1. Parent to Child Communication:
- The `AccountComponent` passes `accountName` and `accountId` to `ContactComponent` using `@api`.
2. Child to Parent Communication:
- `ContactComponent` dispatches a `contactevent` with updated contact details.
- `AccountComponent` listens to this event and handles the data.
This approach ensures seamless communication between the `Account` and `Contact` components in Salesforce LWC while adhering to best practices.
Comments